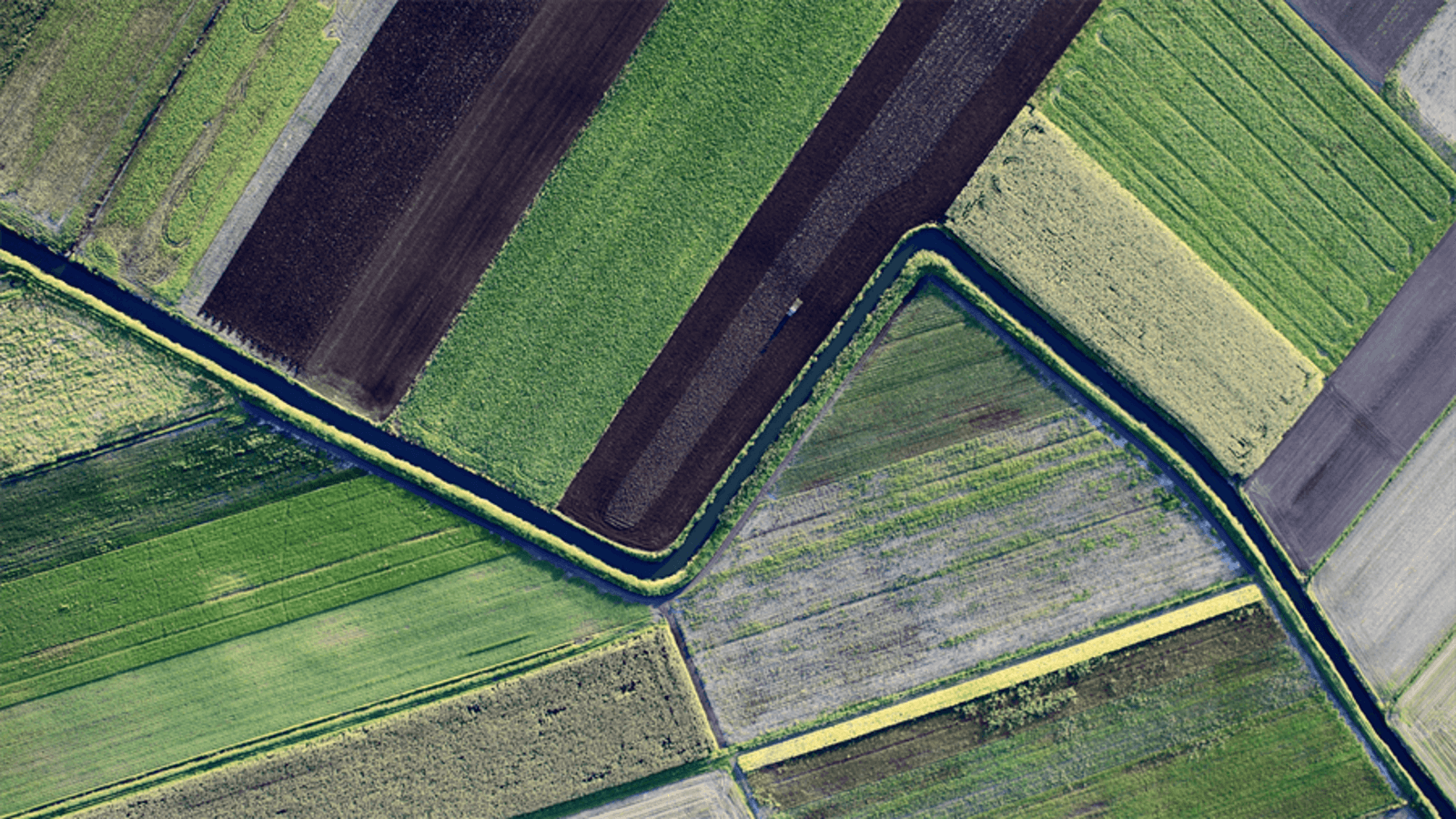
Imagine you are a smart agriculture company that helps farmers optimize their crop yield using IoT sensors. You sell various sensors that can monitor soil moisture, temperature, humidity, and light levels in the farm fields. To help farmers make the most of their resources, you want to build a centralized system. This system collects sensor data from all the fields and provides real-time insights into the health and growth of the crops. With this data, farmers can make informed decisions about when to irrigate, fertilize, and harvest their crops. Using Neo4j, a graph database technology, can be the key to unlocking valuable insights from the collected data. It also enables the smart agriculture system to reach its full potential. In this blog post, we explore how Neo4j can be used to build a powerful smart agriculture system. We start by discussing what Neo4j is and why it is ideal for storing and querying complex, interconnected data. Then, we dive into the specific use cases for Neo4j in smart agriculture, such as identifying crop patterns, predicting crop yields, and optimizing resource usage. Finally, we walk through a step-by-step guide to building a Neo4j-based smart agriculture system that can help farmers make better decisions and improve their crop yield.
Architecture
Hardware components
Software components
Step 1: Preparing your Jetson Nano SD card image for OS installation
To flash the Jetson Nano SD card image to an SD card, you can follow these steps:
- Download Jetson Nano SD card image from NVIDIA.
- Insert the SD card into your computer’s SD card reader.
- Download and install the Etcher software from https://www.balena.io/etcher/.
- Open Etcher and select the Jetson Nano SD card image file you want to flash.
Step 2: Wiring your sensors
The BME680 sensor from Seeed Studio is a compact environmental sensor designed for use in mobile applications and wearables. It can measure temperature, pressure, humidity, and indoor air quality with high accuracy, and is designed to consume very low power. The sensor is compatible with popular microcontroller platforms, such as Raspberry Pi and Arduino, making it easy to integrate into projects.
The Grove Base Hat is a 40-pin Grove add-on board designed to be compatible with Raspberry Pi boards. However, it can also be used with the NVIDIA Jetson Nano by connecting it to the appropriate GPIO pins. It has 15 Grove connectors including 6 digital, 4 analog, 3 I2C, 1 UART, and 1 PWM. This hardware module provides a simple and convenient way to connect Grove sensors and actuators to Jetson Nano. It supports a wide range of Grove modules, such as sensors for temperature, humidity, light, and sound, as well as actuators like motors and displays.
To connect the Grove Base Hat to the Jetson Nano, you must connect the following pins:
- VCC to 3.3V pin on the Jetson Nano
- GND to GND pin on the Jetson Nano
- SDA to pin 3 on the Jetson Nano (SDA1)
- SCL to pin 5 on the Jetson Nano (SCL1)
Once you have connected the Grove Base Hat to the Jetson Nano, you can start using Grove sensors and actuators with your Jetson Nano projects. After wiring the sensors to the Grove, it is recommended to run I2C detection with the i2cdetect command to verify that you see the device: in our case, it shows 76. Please note that the sensor communicates with a microcontroller using I2C or SPI communication protocols.
$ i2cdetect -r -y 1
0 1 2 3 4 5 6 7 8 9 a b c d e f
00: — — — — — — — — — — — — —
10: — — — — — — — — — — — — — — — —
20: — — — — — — — — — — — — — — — —
30: — — — — — — — — — — — — — — — —
40: — — — — — — — — — — — — — — — —
50: — — — — — — — — — — — — — — — —
60: — — — — — — — — — — — — — — — —
70: — — — — — — 76 —
Step 3: Writing a Python code to fetch sensor values
Here is a Python script to fetch sensor values from BME680 sensor:
from bme680 import BME680
# Initialize BME680 sensor object
sensor = BME680()
# Check sensor is connected
if not sensor.begin():
print("Failed to initialize BME680 sensor.")
exit()
# Read and print sensor values
while True:
if sensor.get_sensor_data():
temperature = round(sensor.data.temperature, 2)
humidity = round(sensor.data.humidity, 2)
pressure = round(sensor.data.pressure, 2)
gas_resistance = round(sensor.data.gas_resistance, 2)
print("Temperature: {} C".format(temperature))
print("Humidity: {} %".format(humidity))
print("Pressure: {} hPa".format(pressure))
print("Gas Resistance: {} Ohms".format(gas_resistance))
else:
print("Error reading BME680 sensor data.")
time.sleep(1)
Step 4: Writing a Python program to send BME680 sensor values to the Neo4j graph database
It is time to write Python code to set up a connection to a Neo4j Graph database using the Neo4j driver and BME680 environmental sensor.
from neo4j import GraphDatabase
from bme680 import BME680
import time
# Set up the Neo4j driver
uri = "neo4j+s://41275b2a.databases.neo4j.io"
driver = GraphDatabase.driver(uri, auth=("neo4j", "3DXXXXXXXXXXXXXXXaM"))
# Set up the BME680 sensor
sensor = BME680()
# Define a function to create a sensor reading node in Neo4j
def create_sensor_reading(tx, temperature, humidity, pressure, gas):
tx.run("CREATE (:SensorReading {temperature: $temperature, humidity: $humidity, pressure: $pressure, gas: $gas, timestamp: $timestamp})",
temperature=temperature, humidity=humidity, pressure=pressure, gas=gas, timestamp=int(time.time()))
# Generate and insert sensor readings into Neo4j every 5 seconds
while True:
if sensor.get_sensor_data():
temperature = round(sensor.data.temperature, 2)
humidity = round(sensor.data.humidity, 2)
pressure = round(sensor.data.pressure, 2)
gas = round(sensor.data.gas_resistance, 2)
with driver.session() as session:
session.write_transaction(create_sensor_reading, temperature, humidity, pressure, gas)
print(f"Inserted sensor reading - temperature: {temperature}, humidity: {humidity}, pressure: {pressure}, gas: {gas}")
else:
print("Error reading BME680 sensor data.")
time.sleep(5)
This Python code sets up a connection to a Neo4j graph database using the Neo4j driver and a BME680 environmental sensor. It then defines a function to create a sensor reading node in the graph database with the current temperature, humidity, pressure, gas resistance, and timestamp. The main loop of the code repeatedly generates sensor readings every 5 seconds and inserts them into the graph database using the defined function.
The from neo4j import GraphDatabase
statement imports the Neo4j driver, which allows Python code to interact with a Neo4j database. The from bme680 import BME680
statement imports the BME680 sensor library, which